Javascript Chatgpt Library
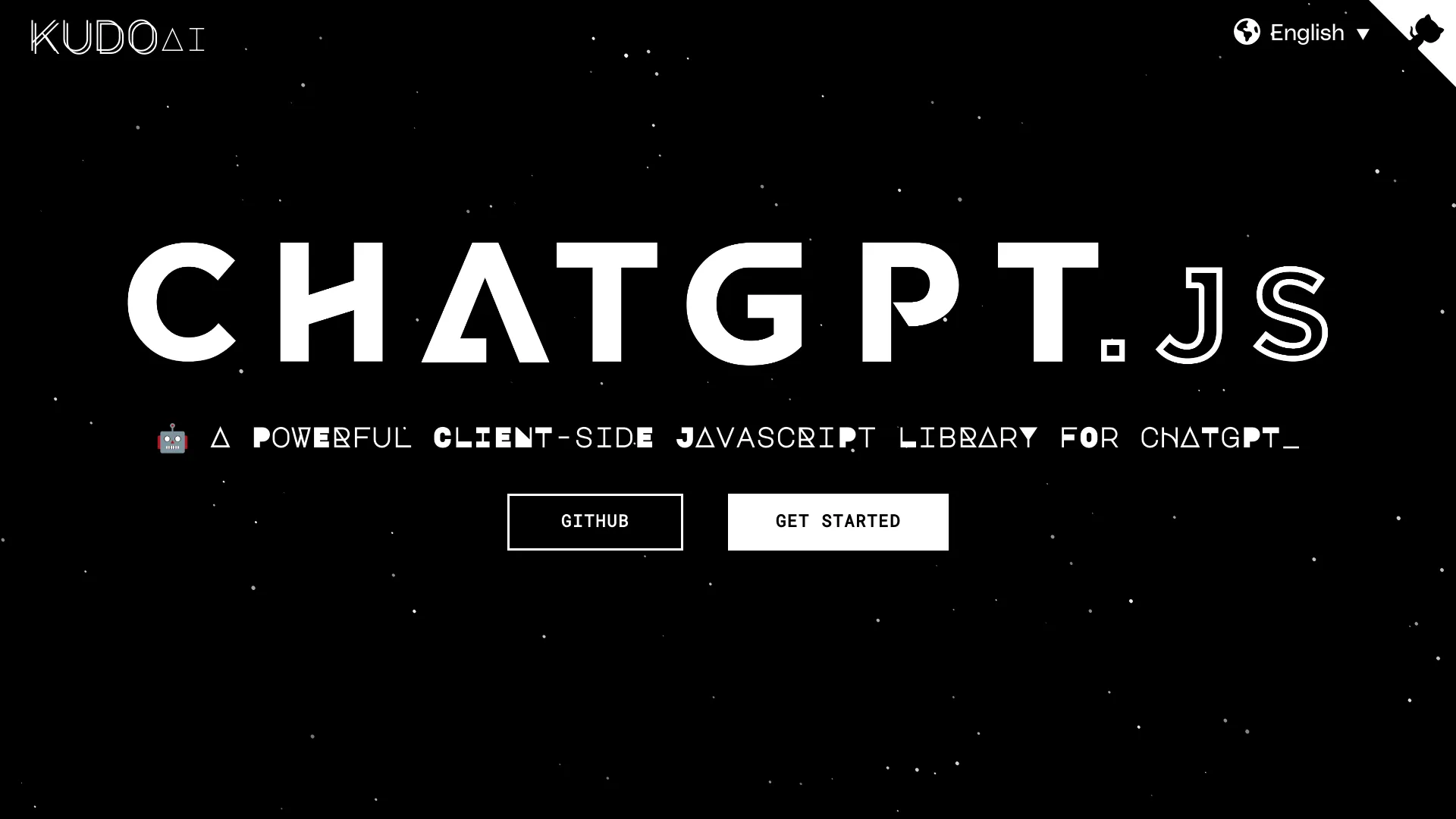
How can I import the ChatGPT JS library into my project?
Importing the ChatGPT JS library can be done in several ways depending on your environment:
For ES11 (2020), you can use the following asynchronous function:
```javascript
(async () => {
await import('https://cdn.jsdelivr.net/npm/@kudoai/chatgpt.js@3.3.5/dist/chatgpt.min.js');
// Your code here...
})();
```For ES5 (2009), you can use XMLHttpRequest:
```javascript
var xhr = new XMLHttpRequest();
xhr.open('GET', 'https://cdn.jsdelivr.net/npm/@kudoai/chatgpt.js@3.3.5/dist/chatgpt.min.js');
xhr.onload = function () {
if (xhr.status === 200) {
var chatgptJS = document.createElement('script');
chatgptJS.textContent = xhr.responseText;
document.head.append(chatgptJS);
yourCode();
}
};
xhr.send();function yourCode() {
// Your code here...
}
```For Greasemonkey and Chrome, you can follow the special instructions provided to ensure compatibility with these platforms.
What are some tools made with ChatGPT JS?
Several tools have been developed using the ChatGPT JS library, showcasing its versatility:
- AmazonGPT: Integrates AI into Amazon shopping for enhanced user experience.
- Autoclear ChatGPT History: Automatically clears your ChatGPT query history to maintain privacy.
- BraveGPT: Adds AI-enhanced answers to Brave Search using GPT technology.
- ChatGPT Auto-Continue: Automates the continuation of generating multiple ChatGPT responses.
- ChatGPT Auto-Talk: Allows for auto-playing of ChatGPT responses.
- ChatGPT Auto Refresh: Keeps ChatGPT sessions fresh to prevent network errors and Cloudflare checks.
- DuckDuckGPT: Adds AI-powered answers to DuckDuckGo Search.
- GoogleGPT: Enhances Google Search with AI answers.
- ThunderAI: Uses ChatGPT in Thunderbird to improve email creation.
How can I customize ChatGPT JS locally using npm?
To customize ChatGPT JS locally, you should download the library via npm by running the following command in your project's root:
npm install @kudoai/chatgpt.js
After installation, you can navigate to node_modules/@kudoai/chatgpt.js
in your project to access and modify the library source as needed. This method allows for full customization and integration into your project workflow.